
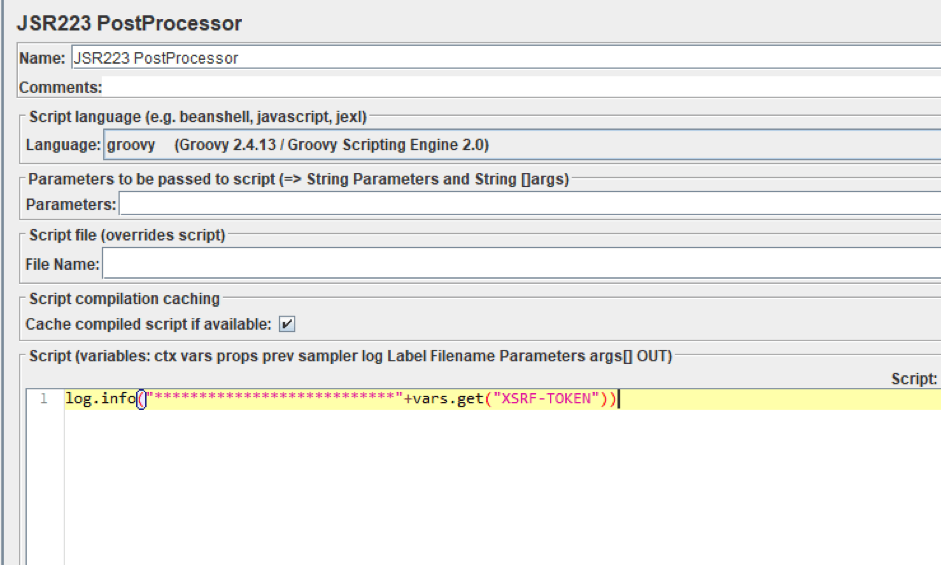
And dealing with a regular expression for a simple replacement of strings is overwhelming.įinally, the new string method string.replaceAll(search, replaceWith) replaces all string occurrences. Unfortunately, you cannot easily generate regular expressions from a string at runtime, because the special characters of regular expressions have to be escaped. This approach works, but it's hacky.Īnother approach is to use string.replace(/SEARCH/g, replaceWith) with a regular expression having the global flag enabled. The primitive approach to replace all occurrences is to split the string into chunks by the search string, the join back the string placing the replace string between chunks: string.split(search).join(replaceWith). If search argument is a non-global regular expression, then replaceAll() throws a TypeError exception.If search argument is a string, replaceAll() replaces all occurrences of search with replaceWith, while replace() only the first occurence.The string methods replaceAll(search, replaceWith) and replace(search, replaceWith) work the same way, expect 2 things: 3.1 The difference between replaceAll() and replace() Note that currently, the method support in browsers is limited, and you might require a polyfill. String.replaceAll(search, replaceWith) is the best way to replace all string occurrences in a string So, the basic of Regular Expression in Javascript is over.įinally, Javascript Regular Expressions Example Tutorial is over.'duck duck go'.replaceAll(' ', '-') replaces all occurrences of ' ' string with '-'. server.jsĬonsole.log(re.test('APP10app')) // true server.jsĬonsole.log(re.test('APPDIVIDEND')) // falseĪlso, the regular expressions can be combined so that we can make it above example true. server.jsĬonsole.log(re.test('appdividend')) // trueīut the following give you falsely result. Instead of matching the particular string, you can choose to match any character in a range. server.jsĬonsole.log(re.exec('you can browse appdividend')) If no match is found, then it returns null. It searches the string for a specified pattern and returns a found text.
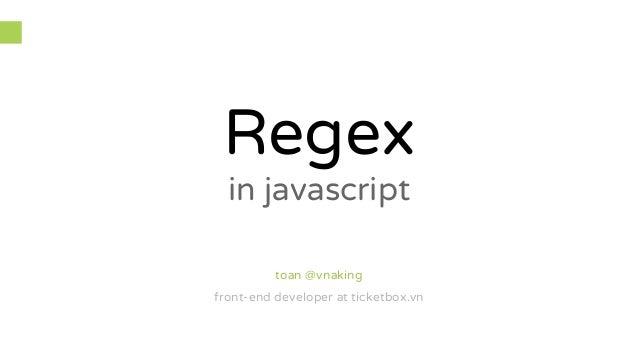
The following example searches the string for the character “ appdividend“: // server.jsĬonsole.log(re.test('you can browse appdividend')) // true #Using exec() methodĪn exec() method is the RegExp expression method. It searches the string for the pattern, and returns true or false, depending on a result. You can test a regex using RegExp.test(), which returns the boolean:Ī test() method is the RegExp expression method. In the literal form it’s delimited by the forward slashes, while with the object constructor, it’s not. In the example above, the appdividend is called the pattern. The second is using a regular expression literal form: // server.js The first is by instantiating the new RegExp object using a constructor: // server.js In JavaScript, a regular expression is an object, which can be defined in the following two ways. Metacharacters are characters with a special meaning: Metacharacterįind a match at the beginning of the end of a wordįind a Unicode character specified by a hexadecimal number xxxx server.jsĬonst replaced = str.replace(/appdividend/, 'youtube') Ĭonsole.log(replaced) #Regular Expression Patterns Expressionįind any of the characters between the bracketsįind any of the digits between the bracketsįind any of the alternatives separated with | You can also do the same replace operation using the Regular Expression. Here, you will get the output like let us browse. server.jsĬonst replaced = str.replace('appdividend', 'youtube') Javascript replace() method replaces the specified value with another value in the string. In the above case also, we will get the same output. We can also search the string with a Regular Expression. Let us see the following example.

The search() method searches the string for a specified value and returns the position of the match. In our case it is appdividend, so it will return the starting position of the appdividend which is 14. Here, what we have done is looking for the starting index of the provided string.
